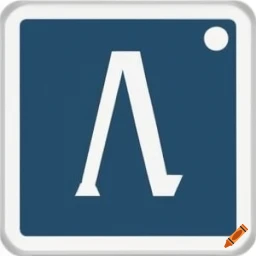
Introduction to C#
C# is a general-purpose, object-oriented programming language developed by Microsoft. It is widely used for building various types of applications, including desktop, web, and mobile applications.
Hello World Example
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
Data Types
C# provides several built-in data types, including:
- int: used to store whole numbers
- double: used to store floating-point numbers
- string: used to store text
- bool: used to store boolean values (true or false)
Control Structures
C# includes various control structures for decision-making and looping:
- if statement: executes a block of code if a certain condition is true
- for loop: repeats a block of code a specified number of times
- while loop: repeats a block of code while a certain condition is true
Functions
In C#, you can define functions to encapsulate reusable blocks of code:
using System;
class Program
{
static void Main()
{
int result = Add(3, 5);
Console.WriteLine("The result is: " + result);
}
static int Add(int a, int b)
{
return a + b;
}
}
Conclusion
This was a brief introduction to C# and some of its basic concepts. C# has a rich set of features that allow you to build powerful and efficient applications. Explore further to learn more!